Why use linter and code analysis tools?
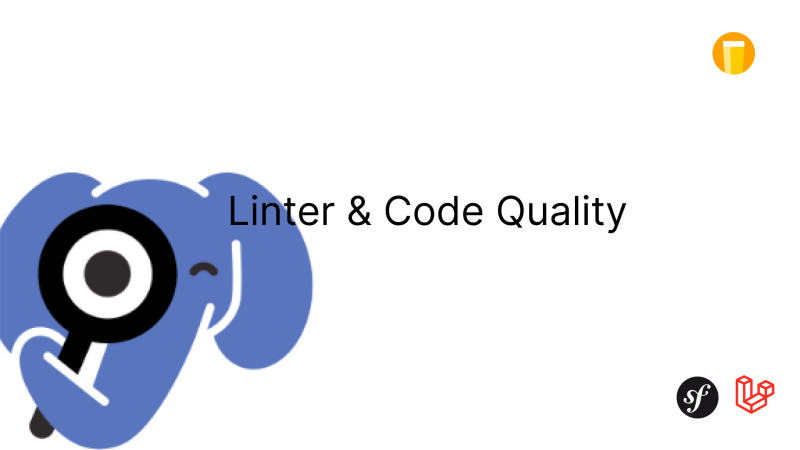
Categories
Symfony Php LaravelWhy I Recommend Using Linters and Scanners to Improve Code Quality
We'll take the example of a PHP application with the Symfony or Laravel framework, but this applies to other languages as well.
What are Linters and Code Scanners?
- A linter allows you to have uniformity, better maintainability, and improved readability.
- A code scanner increases the reliability of your code. Taking PHP as an example, we generally use PHPStan, which enables strong typing, checks if variables are null, etc. We'll look at several examples.
How to Configure Them
Configuring our Linter
With Laravel, we can use a bundle called Laravel Pint
, which provides a basic configuration and simplicity for setup.
Symfony and other frameworks don't have this luxury yet; they use PHP-CS-Fixer
, which is a bit more complex to configure but not insurmountable, especially with today's open-source examples.
// PHP-CS-Fixer
declare(strict_types=1);
$finder = (new PhpCsFixer\Finder())
->in(__DIR__)
->exclude(['var', 'lib'])
;
return (new PhpCsFixer\Config())
->setParallelConfig(PhpCsFixer\Runner\Parallel\ParallelConfigFactory::detect())
->setRules([
'@Symfony' => true,
// customized rules:
'concat_space' => ['spacing' => 'one'],
'phpdoc_align' => ['align' => 'left'],
'phpdoc_separation' => ['skip_unlisted_annotations' => true],
'nullable_type_declaration_for_default_null_value' => true,
'yoda_style' => false,
'phpdoc_to_comment' => false,
])
->setFinder($finder);
Configuring PHPStan
Install PHPStan:
composer require --dev phpstan/phpstan
- Create a file to configure it:
phpstan.neon
. - Add the default configuration.
For all frameworks, we have rules that are already prepared.
# Laravel
includes:
- ./vendor/larastan/larastan/extension.neon
# Symfony
includes:
- vendor/phpstan/phpstan-doctrine/extension.neon
- vendor/phpstan/phpstan-symfony/extension.neon
parameters:
level: 8 # 1 -> 10
parallel:
maximumNumberOfProcesses: 1
paths:
- app
excludePaths:
# add all files/folders you don't want to include
- ./.github
- ./.scripts
- ./docker
- ./node_modules
- ./public
- ./vendor
ignoreErrors:
Running Our Tools
For better convenience, I recommend using make
, which will allow us to avoid memorizing commands.
- Create a
Makefile
- Add these commands
.PHONY: help
.DEFAULT_GOAL = help
## ββ Tools π οΈοΈ βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ
.PHONY: cs
cs: ## code style check
./vendor/bin/php-cs-fixer fix --dry-run --diff
.PHONY: fix
fix: ## code style fix
./vendor/bin/php-cs-fixer fix
.PHONY: phpstan
phpstan: ## phpstan
vendor/bin/phpstan analyse --memory-limit=2G
## ββ Others π οΈοΈ βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ
help: ## listing command
@grep -E '(^[a-zA-Z0-9_-]+:.*?##.*$$)|(^##)' $(MAKEFILE_LIST) | awk 'BEGIN {FS = ":.*?## "}{printf "\033[32m%-30s\033[0m %s\n", $$1, $$2}' | sed -e 's/\[32m##/[33m/'
Running the Linter
To see the modifications that PHP-CS-Fixer
proposes:
make cs
If you're satisfied with the changes, you can apply them:
make fix
Conclusion
These tools ensure that you have quality code while maintaining project maintainability. At first, it's disorienting, but over time you learn a lot, and after 3-4 months, you don't even think about it anymoreβyou do it naturally. So, I believe it's really an asset for your project :)